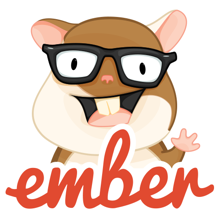
Uploading a file in an Ember test
For the longest time I didn't think it was possible to upload a file in an Ember integration test or acceptance test because clicking on a…
For the longest time I didn't think it was possible to upload a file in an Ember integration test or acceptance test because clicking on a…
To create a Laravel app with Sail, we run the following command in Terminal: I can never remember this so I always have to find this…
Let's say we have a component that yields out block params, and we want to write tests to check those block params. One way to do this would…
Ember Changeset supports nested data. This is documented on the repo. For example: However, what isn't documented at the time of this…
This post covers how to wire up CodeMirror version 5 in an Ember application. First, let's update the file to include the CodeMirror CSS…
This post covers how I used the contenteditable attribute in React and worked around a jumping cursor issue.
This post covers how I used the attribute in Ember and worked around a jumping cursor issue. My First Implementation with a Jumping Cursor…
Recently I was exploring alternatives to Google Analytics and I came across Plausible, which markets itself as lightweight and open source…
In this post, I cover how I used a modifier to set the indeterminate state of a checkbox in an Ember app.
In this post, I cover how I wrote a custom ESLint rule to enforce consistent placement of the @action decorator in an Ember application.
In this post, I cover how I used a custom ember-template-lint rule to address an accessibility issue in an Ember application.
Fetch requests don't have a timeout, but we can make them time out using Promise.race() and AbortController.
Did you know that the router service in Ember provides everything we need to recreate the LinkTo component? Let's create a component called Link with the same public API as LinkTo.
Recently I learned that we can provide an autocomplete feature for input elements natively in the browser via the datalist tag. I started playing around with it and made a reusable Glimmer component to search against any...
Recently I learned about server-sent events, which allow for a server to send new data to the browser at any time by pushing messages over a…
Recently I was trying to figure out how to prevent a deeply nested object from being mutated. That is, I didn't want properties in the…
Recently I was working on making a modal more accessibile. When I opened the modal and tried to use the keyboard to navigate, the focus…
In this post, I cover how to get up and running with PostCSS using PostCSS CLI and go over popular plugins including postcss-import…
Recently I was helping someone make all modals in an application close when a user presses the escape key. Thankfully, ember-keyboard makes…
This post is based off a question I had asked in the Ember Discuss forum about the caching of getters. Recently I discovered that getters…
Are you working on an Ember project using Emblem templates and want to convert to Handlebars? This post covers my approach and experience.
This post covers an example of migrating a computed property to a native class getter and addresses a few questions that I had along the way.
If you're in the midst of transitioning your classic classes to native classes, it may be helpful to know that you can still use the @action and @tracked decorators.
ES2020 brings the nullish coalescing operator to JavaScript, which allows us to create default values succinctly.
Does recursion make your head spin? Haven't used it in awhile and want a refresher? If so, this series is for you.
Learn how to abort fetch requests with the AbortController class and how to do it in a React component.
Learn how to sprinkle in Svelte 3 components into a Jekyll site.
This post shows how to run a Jekyll site with Docker.
This post covers how to use SQLite with PHP on Heroku.
This post shows how to create a JSON feed of all of your posts in a Jekyll site.
Learn how to sprinkle in Svelte 3 components into an existing project.
Learn how to set up continuous integration with GitHub Actions for your Ember CLI project.
Installing PHP on Windows
Learn how to deploy your Create React App project to Surge.sh.
An introduction to JavaScript Decorators on methods in ES6 classes.
This post is a brief introduction to protecting web applications against Cross-Site Request Forgery Attacks (CSRF).
This post covers how to deal with foreign key type of attributes in an API with Ember Data.
This post covers how I used embedded records with a JSON:API-ish response.
The URLSearchParams and URL classes can be used to build and parse query strings in JavaScript in both the browser and Node.
This post covers how to build a simple chat application with Web Sockets in Node.js.
Does recursion make your head spin? Haven't used it in awhile and want a refresher? If so, this series is for you.
Does recursion make your head spin? Haven't used it in awhile and want a refresher? If so, this series is for you.
Does recursion make your head spin? Haven't used it in awhile and want a refresher? If so, this series is for you.
Does recursion make your head spin? Haven't used it in awhile and want a refresher? If so, this series is for you.
This post covers two new methods in ES2019 for flattening JavaScript arrays, flat and flatMap.
This post covers a problem I ran into when reading files on Heroku in an Elixir Phoenix app.
This post covers a few things I learned when decoding JSON into Elixir structs.
In this post, we'll look at how to loop in Elixir through recursion by rebuilding Enum.each, Enum.reduce, Enum.map, and Enum.filter.
In this post, we're going to look at capturing functions and expressions, which can make Elixir code more concise.
Time to dive into functional programming and learn Elixir. This post covers keyword lists.
This post covers new Ember component features as of 3.4.
This post covers creating custom QUnit assertions in Ember.
This post covers how I handled tracking hasMany relationship changes with ember-changeset.
This post covers how to access the instance of a model in the defaultValue function of an attribute to conditionally set a default value.
This post covers how to import files from node_modules in Ember
This post covers how to extract metadata from a custom API with Ember Data.
Contextual Components are one of my favorite features in Ember. In this post, I show how you can implement the same pattern in React components using the Render Props pattern.
In this blog post, I cover the Render Props pattern in React and what it looks like in Ember.
This post covers how I approached conditional validations with ember-changeset.
Deploying Laravel with SQLite to Heroku
The fundamentals of SQLite
In this post, I cover how to test with relative dates and timezones in an Ember application.
In this post, I cover how you can subclass an array in JavaScript in ES2015 (ES6).
This post explores a few approaches to mocking services in acceptance tests and shows an example that stubs window.confirm.
Client-side templating with Handlebars.js
Event delegation examples using jQuery
In this post, I go over the iterable and iterator protocols and show some examples of making your own objects iterable so that they can be used with the for...of loop or the spread operator.
This post is an introduction to the Set class in JavaScript.
The fundamentals of constructor functions and ES6 classes
Examples of using the Google Maps JavaScript API
The fundamentals of objects in JavaScript
How I built a checkbox list Ember component using contextual components and an ES 2015 Set
In my new SitePoint course "Test-Driven Development in Node.js", I teach test-driven development using practical examples, covering a range of topics including the fundamental theory, testing asynchronous code, and the d...
Imagine you have the following component: where looks like: and the template looks like: The component is a light wrapper around the…
One technique I often like to do when I am refactoring is move relationship logic outside of an action into the Ember Data model behind a custom method. Let me show you.
One idea I've been been experimenting with in an Angular 1.5 application I've been working on is using ES6 generators to manage concurrency in components.
Have you ever felt like you had a gap in your learning because you didn't know common algorithms and data structures in computer science? Maybe you've tanked an interview where they asked you an algorithm question? Want ...
In Ember Data, if you delete a record from the store, related records are kept in the store. How do you cascade delete related records? Find out in this post.
Did you know that JSON:API supports updating relationships independently at URLs from relationship links? Learn how to do this with Ember Data.
This post covers what are generator functions in ES6, how generator functions work, how generators and promises work together, and some examples of generator functions used in other libraries and frameworks.
Have you ever argued with your team on how an API should be formatted? JSON:API was created to standardize how APIs are built and keep API formatting discussions to a minimum.
The Page Object design pattern is used to isolate HTML structure and CSS selectors from your tests. In this post and video tutorial, I'll refactor an acceptance and integration test in Ember to use a page object with the...
If you're new to JavaScript, you may be wondering what the difference is between using a classic `for` loop versus using the `forEach()` method on arrays. In this post, I'd like to discuss why you might want to choose on...
A podcast where three JavaScript developers discuss strategies and tips to help your career as a software developer.
Ever wondered how to create your own custom Ember CLI command? Recently I went through this process and today I'd like to share what I learned in case you wanted to create your own custom command too.
One of the challenges of working with dates is unit testing code that depends on the current date. Let's look at how to stub out "today".
Have a custom API that you aren't sure how to use with Ember Data? Interested in writing your own adapter or serializer? Want to just know more about how Ember Data works? This is the Ember Data book you have been waitin...
Get up and running with Ember FastBoot with this video tutorial. This video tutorial goes through converting a simple application using jQuery AJAX calls, components, and Ember Data to using Ember FastBoot in under 5 min...
This post shows how you can style your select menus with modern CSS
TL;DR - If both success and reject handlers are executing unintentionally, you might be forgetting to throw after you catch in the promise chain.
If you've had to work with Ember Data and non-standard APIs, you may have dug into the adapters and serializers a bit and seen snapshot as a parameter to a few of the methods. Let me show you what a snapshot is and why y...
Many APIs use nested resource paths. That is, URL paths that contain a hierarchy of resource types. How do you handle that in Ember Data? Let me show you.
Here are the slides from my presentation at the EmberSC meetup on 2/17/16 on Ember Data and Custom APIs.
One pain point of Ember that I often hear is that the learning curve is a little steep. Because Angular is extremely popular, I'd like to introduce Ember from the perspective of an Angular developer, hopefully getting th...
Not too long ago I wrote about Testing Serializers in Ember. The examples in the post went over testing the serialization process on responses and verifying methods like normalizeResponse and keyForRelationship worked co...
In today's post, I'd like to share a few ways of how you can work with nested data in Ember Data models.
Have you ever been on a project where you make one change and later realize you broke something else? It feels like you're playing whack-a-mole. In today's post, I'd like to introduce you to some of the benefits of unit ...
When I first started working with Ember Data in the 1.X days, one of the most frustrating things was having to work with custom APIs. In this post, I'd like to share a few common ways to customize serializers that others...
In this post, we'll look at how integration tests can be used in conjunction with unit tests to effectively test different aspects of your Ember components.
Interested in giving Ember a try but don't want to install Ember CLI on your machine? Or maybe you love Cloud 9 IDE but are unsure of how to run Ember CLI on there. Check out this short video I created that walks you thr...
This post covers what Ember Data expects for error responses and how they map to our model attributes.
In today's post, I want to show you how a fluent interface, also known as a fluent API, can help improve the readability of your code.
When working with Jasmine, you might find yourself wanting to control which tests execute. Let me show you how to do this with Jasmine.
A serializer in Ember Data is used to normalize response payloads and serialize request payloads as data is transferred between the client and the server. This post covers the serializers that ship with Ember Data.
For those who want to start learning Ember 2, here is a list of my recommendations for the best places to learn Ember.
Ember Data has a feature called transforms that allow you to transform values before they are set on a model or sent back to the server. If you've been working with Ember Data, then you have already been using transforms...
This session will introduce you to Ember, a framework for building ambitious web applications. You will build an application using Ember 2 and its companion command line utility Ember CLI. We'll use the power of Ember Da...
How do you go about testing controllers, components, and services that are given Ember Data objects like DS.AdapterPopulatedRecordArray or DS.RecordArray? Let me show you!
How do you go about testing methods on serializers like normalizeResponse and keyForRelationship? Let me show you!
The adapter design pattern is very useful in JavaScript when it comes to data fetching and persistence. The travel adapter is the most…
Earlier this year I wrote about Unit Testing Angular Directives with Isolate Scope. Now that I am working with Angular again, I've…
Rapid Ember.js is an excellent 60 minute introduction to learning the Ember.js framework. The author William Hart does a great job at…
Lately I have been trying out more end-to-end / integration testing with CasperJS. Having these tests in place seems valuable for critical…
Writing unit tests when working with Require.js is tough. Out of the box, Require.js does not make mocking very easy. It involves setting up…
As a frontend developer, you might find yourself in a situation where you have to work with unRESTful endpoints. If you are working with…
When it comes to testing in JavaScript, I would say that there are two types of tests: unit tests and end to end tests, which are also…
In this post, learn how to transform code using forEach to using reduce on JavaScript Arrays.
If you are building complex user interfaces, you will at some point want to start unit testing your directives. Below is a simple directive…
Custom promises can really clean up your code in certain situations. For me, I have found that using custom promises can clean up consumers…
I get asked pretty frequently, "What is the best way to learn Angular.js?". Here is a path of resources in order of what I think is a good…
Recently I upgraded the unit tests for a work project from using Jasmine 1.3 to Jasmine 2.1. The suite had about 400 tests. When I upgraded…
In Angular, we can mock out services using $provide. Let's look at a simple example. Here we have two services using the API in our module…
Often times in web applications you only want a single instance of a class/constructor/reference type to ever be created. This is called the…
Getting started with unit testing your Angular code can be tricky due to setting up your test runner, learning some of the conventions, and…
Testing in JavaScript is becoming expected by developers more and more. But where do you start? There are so many framework choices out…
There are many things that you need to consider when writing JavaScript for an international site. Things such as formatting currency, dates…
One of the difficult parts I am experiencing when working with Backbone is separating out display logic properties from models. Let's look…
Last year I started a new job as where I am currently tasked with rebuilding an ecommerce site for the company. The JavaScript stack is…
Modules allow us to organize code and prevent polluting the global namespace. Until ES6, JavaScript didn't have a native module system…
Over the past year I have been reading a lot about design patterns. One of the patterns I learned about was the Template Method pattern…
CoffeeScript has been an interesting language to learn. At first, I wasn't really into it because it required me to learn another another…
One of the things I have found most challenging when working with Backbone.js is writing maintainable and unit testable views. Based on…
Recently I gave angular-data a try and found it awesome for data modeling in Angular.js. Why use angular-data? There are many ways to model…
When it comes to memory management and JavaScript applications using Backbone, you'll often hear about zombie views and how easy it is to…
Learning how to manage asynchronous code in JavaScript can be challenging. In the browser, asynchronous operations come in the form of AJAX…
In the [last post on Request Expectations]({% post_url 2014-01-18-request-expectations-with-httpBackend %}), we looked at how we can set…
So you have an Angular service that communicates with an API, but you're not really sure how to go about testing it. Maybe you read the…